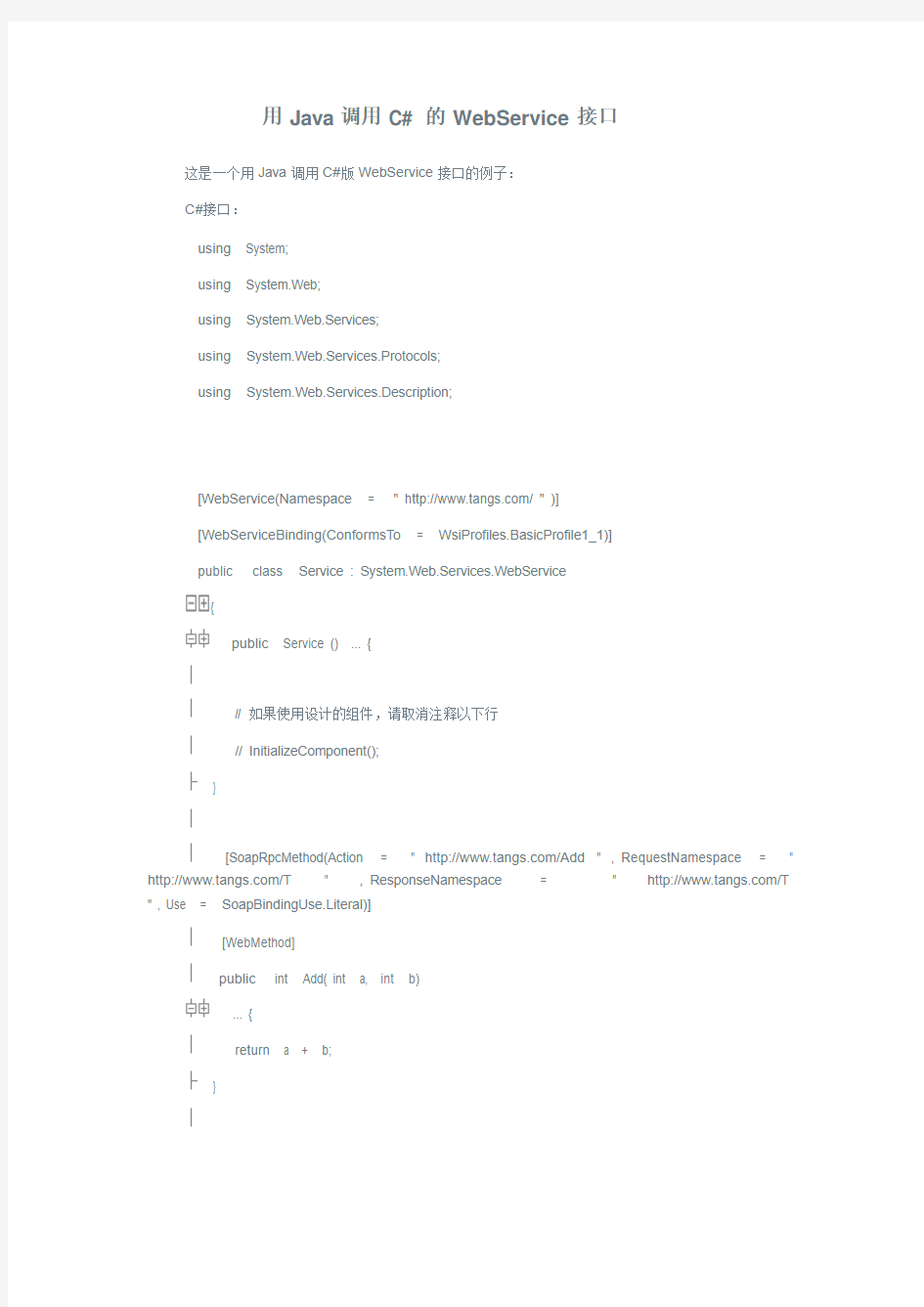
用Java调用C# 的WebService接口
- 格式:doc
- 大小:119.00 KB
- 文档页数:4
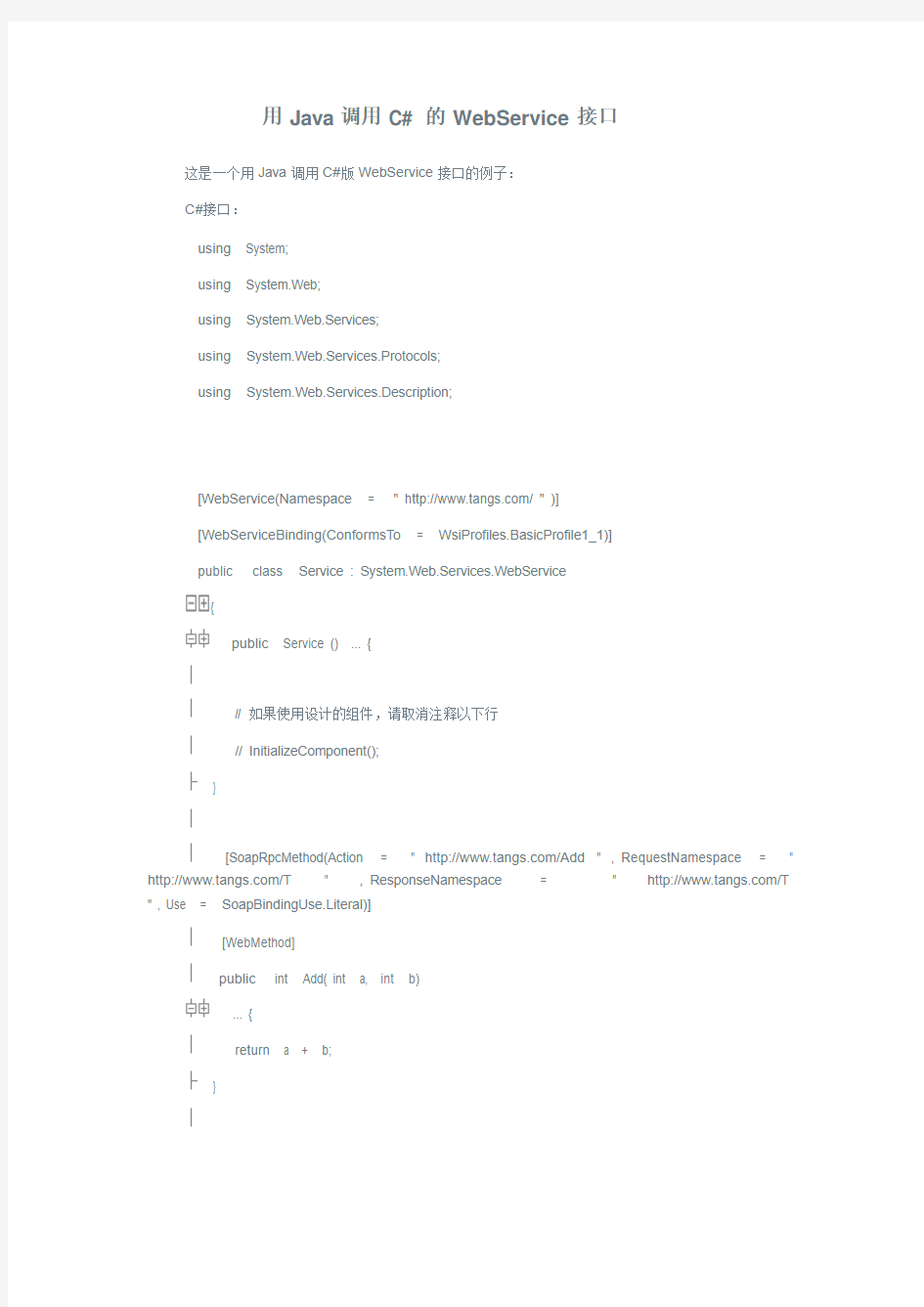
用Java调用C# 的WebService接口
这是一个用Java调用C#版WebService接口的例子:
C#接口:
using System;
using System.Web;
using System.Web.Services;
using System.Web.Services.Protocols;
using System.Web.Services.Description;
[WebService(Namespace = " / " )]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
public class Service : System.Web.Services.WebService
{
public Service () ... {
// 如果使用设计的组件,请取消注释以下行
// InitializeComponent();
}
[SoapRpcMethod(Action = " /Add " , RequestNamespace = " /T " , ResponseNamespace = " /T " , Use = SoapBindingUse.Literal)]
[WebMethod]
public int Add( int a, int b)
... {
return a + b;
}
[SoapRpcMethod(Action = " /Hello " , RequestNamespace = " /T " , ResponseNamespace = " /T " , Use = SoapBindingUse.Literal)]
[WebMethod]
public String HelloWorld()
... {
return " Hello, world! " ;
}
}
...
Java调用这个Webservice中的Add方法和HelloWorld方法:
1.有参方法:Add
public static void addTest() {
try ... {
Integer i = 1 ;
Integer j = 2 ;
// WebService URL
String service_url = " http://localhost:4079/ws/Service.asmx " ;
Service service = new Service();
Call call = (Call) service.createCall();
call.setTargetEndpointAddress( new .URL(service_url));
// 设置要调用的方法
call.setOperationName( new QName( " /T " , " Add " ));
// 该方法需要的参数
call.addParameter( " a " , org.apache.axis.encoding.XMLType.XSD_INT,
javax.xml.rpc.ParameterMode.IN);
call.addParameter( " b " , org.apache.axis.encoding.XMLType.XSD_INT,
javax.xml.rpc.ParameterMode.IN);
// 方法的返回值类型
call.setReturnType(org.apache.axis.encoding.XMLType.XSD_INT);
call.setUseSOAPAction( true );
call.setSOAPActionURI( " /Add " );
// 调用该方法
Integer res = (Integer)call.invoke(
new Object[] ... {
i, j
}
);
System.out.println( " Result: " + res.toString());
} catch (Exception e) ... {
System.err.println(e);
}
} ...
运行,结果返回:Result:3
2.无参方法:HelloWorld
public static void helloTest() {
try ... {
String endpoint = " http://localhost:4079/ws/Service.asmx " ;
Service service = new Service();
Call call = (Call) service.createCall();
call.setTargetEndpointAddress( new .URL(endpoint));
call.setOperationName( new QName( " /T " , " HelloWorld " ));
call.setUseSOAPAction( true );
call.setSOAPActionURI( " /Hello " );
String res = (String)call.invoke(
new Object[] ... {
null
}
);
System.out.println( " Result: " + res);
} catch (Exception e) ... {
System.err.println(e.toString());
}
} ...
可以看到,调用无参的webservice和有参的基本相同,不过无参调用时,不需要调用Call的addParameter方法和setReturnType方法
执行结果返回:Hello, world!